Pointers
Well, it is believe that real power of C lies in the proper use of pointers. However it may cause nightmares, if they are not properly handled.
Pointers is a type of variable which holds the memory location of other variable. A pointer can be incremented/decremented, i.e, to the next/previous memory location.
Pointer Syntax
Here is how we can declare pointers.
int* p;
int *p;
Advantages of Pointers in C
- Pointers are useful for accessing memory locations.
- The purpose of pointer is to save memory space and achieve faster execution time.
- Pointers are used to return multiple value from function.
- Pointers helps in dynamic memory allocation.
- Pointers can be used with array and string to access elements more effeciently.
Difference between Array and Pointer
Array |
Pointers |
An array is a variable and cannot be appear on the left hand side of an assignment operator once it is declared. |
A pointer is a variable which can be appear on the left hand side of an assignment operator after declaration. |
E.g. if a is array name then a = &variable; (invalid) |
E.g. if p is pointer variable then p = &variable; (valid) |
Relationship between Array and Pointer
Array is known as constant pointers since name of the array itself represent the memory location of first element of an array.
So, array is also called constant pointer.
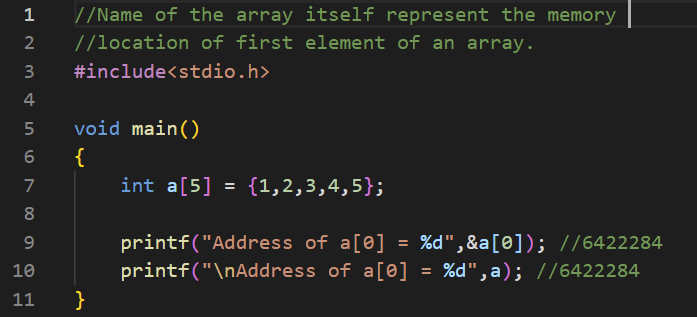
We can access the values and addresses of an array element by following methods
1. Normal Method in 1D Array
2. By using array name itself as a pointer in 1D Array
3. By using pointer variable in 1D Array
1. Normal Method in 2D Array
2. By using array name itself as a pointer in 2D Array
3. By using pointer variable in 2D Array