Character Set
The basic building blocks to form a C programming language is known as Character Set.
So, the character set are the upper case alphabet like A...Z, lower case alphabet like a...z, the digits like 0,1,2...9 and other special syombles like +,-,*,/,?,/ and white spaces are collectively known as C Character set.
These character set are used to build variables, operators, expressions etc.
Keywords
Keywords are the reserved words that have standard and pre-defined meaning.
All keywords are written in lower case alphabet.
All the keywords are identifier but all the identifier are not keywords.
Example: auto, break, if, volatile, double, int, char, const, do, double, long, short, unsigned, void, case, else, default, signed, ada
Data Types
Data types are the keywords that determine the characterstics of variable.
Data Types |
Memory Requirements |
Format Specifier |
Range |
Character |
1 byte |
%c |
unsigned char (0 to 255) |
Integer |
2 bytes |
%d |
signed int (-32,768 to 32,767) unsigned int (0 to 65,535) |
Float |
4 bytes |
%f |
3.4x10^38 to +3.4 x 10^38 |
Double |
8 bytes |
%lf |
1.7 x 10^308 to + 1.7 x 10^308 |
For more format specifier refer to
aticleworld.com
Constants
Constant referes to the value that do not change during program execution.
There are four types of constant in C.
Integer Constant
Integer constant are the whole numbers that does not contain decimal or fractional part.
E.g. 123, 16, 15, 100, 300 etc.
Floating point constant
Floating point constant is the value or number that contain decimal or fractional part.
E.g. 63.3, 1.33, 1.6 etc.
Single Character constant
A single character constant is a single alphabet, digit or symbol enclosed in a single quotation mark.
E.g. ''x','Á', '1', '?
String constant
A string constant is alphabet, digits or symbol enclosed between double quotation mark. The length of string constant cannot be more than 255.
E.g. "cat", "Bat", "Hello", "Ram123"
Variables
Variables refers to the value that can be changed during program execution. Variables are the name given to the location in the memory of the computer.
Rules for declaring the variables:
- Only alphabet, digits and underscore character are allowed.
- Keyword, white space and any special character except underscore are not alloud.
- Upper & lower case alphabet are not interchangable.
- Only first 31 character are significant.
Statements
A statement causes the computer to carry out some action.
Three different classes of statement in C.
Expression statement
An expression statement consist of an expression followed by a semicolon.
E.g. S = 3; x = 10;
Compound statement
A compound statement consists of several individual statements enclosed within a pair of braces {}.
E.g. { length = 2; breadth = 3;}
Control statement
The control statement are used to create spcial program features, such as logical tests, loops and branches.
E.g.
if(a%2==0)
{
printf("The given number is even");
else
printf("The given number is odd");
}
Escape Sequence
The certain non-printing characters, having specific function used in C programming languages is known as scape sequences.
In another words the escape sequence is used to change the format of output and it is always written along with slash.
ESCAPE SEQUENCE |
FUNCTION |
\n |
Used to start next line |
\t |
Used to get horizontal tab |
\a |
Used to get alarm signal |
\b |
Used for backspace |
\r |
Used for carriage return |
\" |
Used for double quote |
\\ |
Use to print Backslash |
\v |
Used to get vertical tab |
Symbolic constant
A symbolic constant is a name that substitude to a sequence of characters. The character may be numeric constant, character constant or string constant.
Thus a symbolic constant allows a name to appear in a place of numeric constant, character constant or string constant.
When a program is compiled, each occurance of a symbolic constant is replaced by its corresponding character sequence.
For E.g.
#define PI 3.1415 where PI is used as symbolic constant whose value is 3.1415
#define ROW 50 where ROW is used as symbolic constant whose value is 50
Preprocessor Directives
- As the name suggests Preprocessor are programs that process our source code before it passes through the compiler.
- And the commands used in preprocessor are called preprocessor directives and they begin with '#' symbol.
- The '#' symbol indicates that, whatever statement starts with #, preprocessor program will execute this statement.
- We can place these preprocessor directives anywhere in our program.
- One of the task of preprocessor is to removes all the comments. Since a comment is written only for humans to understand the code. So, preprocessor removes all of them as they are not required in the execution and won't be executed as well.
There are 4 main types of preprocessor directives:
- Macros Expansion Directive (#define)
- File Inclusion Directive (#include)
- Conditional Directive (#ifdef, #else, #endif)
- Other Directives (#undef, #pragma)
1. Macros Expansion Directive:
A macro is a segment of code which is replaced by the value of macro. Macro is defined by #define directive.
It is called a macro processor because it allows you to define macros, which are brief abbreviations for longer constructs.
Whenever this name is encountered by the compiler the compiler replaces the name with the actual piece of code.
The #define directive is used to define a macro. Note: Macro definition do not need a semi-colon to end.
Macros with arguments
We can also pass arguments to macros. Macros defined with arguments works similarly as functions.
Predefine Macros
Macro |
Description |
_DATE_ |
The current date as "MMM DD YYY" format. |
_TIME_ |
The current time as "HH:MM:SS" format. |
_FILE_ |
This contains the current filename as a string literal. |
_LINE_ |
This contains the current line number as a decimal constant. |
_STDC_ |
Defined as 1 when the compiler compiles with the ANSI standard. |
2. File Inclusion Directive
This type of preprocessor directive tells the compiler to include a file in the source code program. There are two types of files which can be included by the user in the program:
Header File or Standard files:
Header File like #include< stdio.h > and #include< conio.h > are included in this section. The '<' and '>'' brackets tells the compiler to look for the file in standard directory.
User defined files:
When a program becomes very large, it is good practice to divide in into smaller files and include whenever needed. These types of files are user defined files. These files can be included as:
#include"filename"
3. Conditional Directive:
Conditional Compilation directives are type of directives which helps to compile a specific portion of the program or to skip compilation of some specific part of the program based on some conditions. This can be done with the help of two preprocessing commands
'ifdef' and
'endif'.
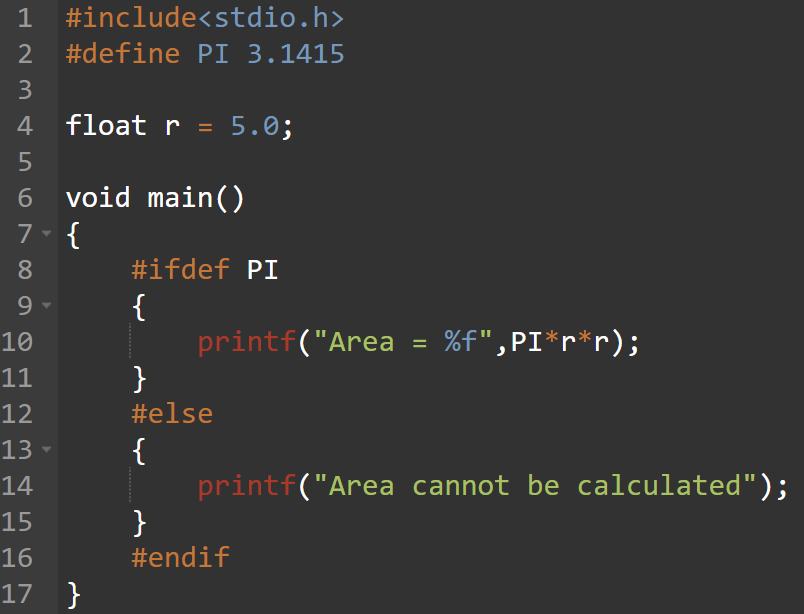
If the macro with name as 'PI' is defined then the block of statements will execute normally but if it is not defined, the compiler will simply skip this block of statements.
4. Other Directives
Apart from the above directives there are two more directives which are not commonly used. These are:
- #undef Directive
- #pragma Directive
-
- #pragma startup and #pragma exit
- #pragma warn Directive
#undef Directive: The #undef directive is used to undefine an existing macro. This directive works as:
Using this statement will undefine the existing macro PI. After this statement every "#ifdef PI" statement will evaluate to false.