Introduction to Structure
An array can be used only to represent a group of data items that belong to the same data type, such as int or char. However, if we want to represent a collection of data items of different types using a single name, array cannot be used. In such a situation, structure is used, which is a method of packing/encapsulating data of different types.
Therefore a structure can be defined as a user-defined data type that enables us to store the collection of different data types, grouped together under a single name. Each element of a structure is called a member.
Defining a Structure
To define a structure, you must use the struct keyword.
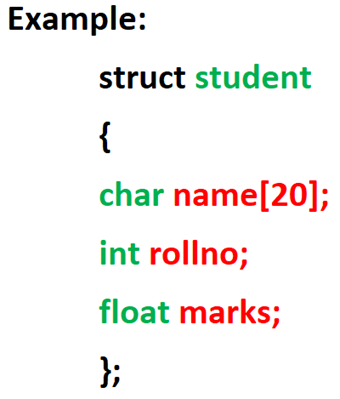
Here, struct is the keyword; student is structure name which is a new data type; name, rollno and marks are the members of the structure.
Declaring structure variable
Once a structure_name is declared as new data type, then variables of that type can be declared in the main function as
Another way to declare structure variables, at the time of defining the structure.
Initialization of structure
To initialize the member of structure first declare the structure with member then create the structure variable and assign the value as
Accessing Member of Structure
The dot ‘.’ operator which is also called member operator connects a structure_variable with a member of the structure.
Array of Structure
An array of structure is nothing but just a representation of structure variable as an array.
Array and Structure
Array |
Structure |
An array is a collection of related data elements of same type. (homogeneous) |
A structure can have elements of different types. (heterogenous) |
An array is a derived data type. |
A structure is a user-defined data type. |
An array behaves like a built-in data types. We can declare an array variable and use it. |
First we have to define a structure and then declare the variables of that type. |
Array name is pointer to the first element of it. |
Structure name is not pointer. |
Structure and Function
Like an ordinary variable, a structure variable can also be passed to a function.
While passing structure variable to a function it is essential to declare structure above the main function as global structure.
We can pass the C structures to functions in 3 ways:
- Passing each item of the structure as a function argument. It is similar to passing normal values as arguments. Although it is easy to implement, we don't use this approach because if the size of a structure is a bit larger, then our life becomes miserable.
- Pass the whole structure as a value.
- Pass the address of the structure (pass by reference).
Pass the whole structure as a value
In this method the entire structure is passed as an argument to a function. Since only a copy of the structure is sent, any changes made to structure members are not reflected into the original structure. It is therefore necessary for the function to return the entire structure back to the calling function.
For Example:
Define a structure student with member name, rollno, marks of student. WAP to enter the information of the structure student and display it using function (Call by value).
Pass the address of the structure (pass by reference)
In this method, the address of the structure is passed to the function. Any changes made to the structure members are reflected on to the original structure. This is because pointers deal direclty with the memory addresses of actual argument.
For Example:
Define a structure student with member name, rollno, marks of student. WAP to enter the information of the structure student and display it using function (call by reference).
Passing an array of structure to a function
Passing an array of structure to a function involves the same syntax and characteristics as passing an array of an ordinary type to a function.
So while passing array of structure to a function, in calling function name of the structure variable (which is an array of structure) is only required.
In called function, name of the structure variable with empty square bracket is required and size is optional.
In passing an array of structure to a function, any changes that are made within the function definition are also visible within the calling function.
Pointer to Structure
We know that pointer is a variable and is used to hold the address of some other variable.
Similary pointer variable can also be used to hold the address of structure variable too.
And the link between structure variable and pointer variable is established using structure pointer operator i.e. '->'.
For Example:
WAP to enter the value of member variable age and weight of a structure person using pointer to structure.
Passing Structure pointer to a function
A structure pointer can be passed to a function. When a pointer to a structure is passed as an argument to a function, the function is called by reference.
Therefore, any changes that are made within the function definition are also visible within the calling function.
For Example:
Define a structure student with member name, rollno, marks of student. WAP to enter the information of the structure student and display it using passing structure pointer to a function.
Nested Structure (Structure within another Structure)
When one structure is declared as the member of another structure then it is called nested structure. The outer structure is known as nesting structure and the inner structure is nested structure. Let us consider a structure date which has members day, month and year. The structure date can be nested within another structure student (i.e. structure date is member of another structure student).
Similarities between Structure and Union
- Both are user-defined data types used to store data of different types as a single unit.
- Their members can be variable of any type, including structures and unions or arrays.
- A structure or a union can be passed by value to functions and returned by value by functions.
- '.' operator is used for accessing members.
- Both structures and unions support only assignment = and sizeof operators.
Difference between Structure and Union
|
Structure |
Union |
1. Keyword |
The Keyword struct is used to define a structure. |
The Keyword union is used to define a union. |
2. Initialization of Members |
Several members of a structure can be initialize at once. |
Only the first member of a union can be initialized. |
3. Accessing Members |
All structure member can be accessed at once. |
All Union member cannot be accessed at once. Only one member can be accessed at a time. |
4. Value Altering |
Altering the value of a member will not affect other members of the structure. |
Altering the value of any of the member will alter other member values. |
5. Memory |
In structure, each member has its own storage location. |
In union, all the member uses the same memory location. |
6. Size |
The amount of memory required to store a structure variable is sum of sizes of all the member. |
Amount of memory required is the size of the largest union member. |
7. Example: |
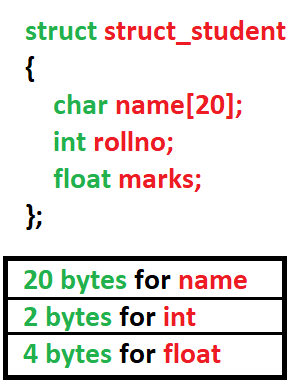 |
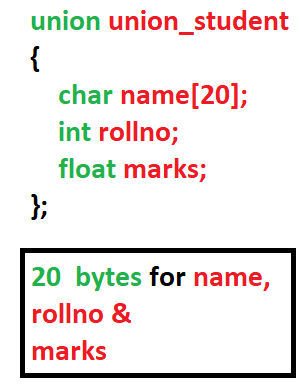 |
8. Total memory allocation |
Total bytes = 20 + 2 + 4 = 26 |
20 bytes are there between name, rollno, and marks because the largest memory occupies by char array which is 20 bytes. |